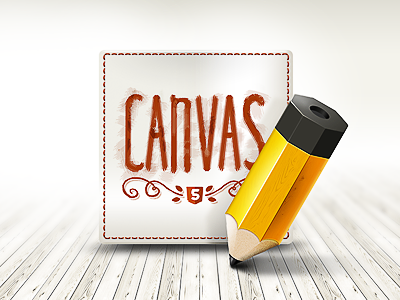
Once we have declared the element in our html file we need to draw something using JS. Here I enlcose some code to draw a line inside the canvas.
function draw() { var canvas = document.getElementById("board"); if (canvas.getContext) { var context = canvas.getContext("2d"); context.beginPath(); context.moveTo(10, 10); context.lineTo(100, 100); context.stroke(); } } window.onload = draw;
We are selecting the canvas element with the first line of our script using the DOM method getElementById(), this why we need an id in our HTML. Then we try to use an HTML5 characteristic to know if the browser is compatible. Then we get the context from canvas in 2 dimensions and we start drawing an stroke. Finally we add this method to the load process.
In a similar way, we can draw other shapes like rectangles by using these three methods:
- fillRect: draw a filled rectangle
- strokeRect: draw only the border of the rectangle
- clearRect: clean a part of the rectangle.
All these methods needs four parameters: x, y, width and height. The coordinates have their origin in the top-left part of the canvas element. Although, the rest of drawing methods used in Canvas 2D need a path: curves, arcs,… For these reason we need to take some extra steps:
In the code we try to draw the classic smile by drawing each part of it. Unfortunately we are drawing over the canvas component like if we are not raising the pencil and it looks like this:
To avoid this effect we can use the method moveTo. With moveTo we raise the pencil over the canvas and we can get the effect of the following image.
Here, more methods to draw curves:
Finally I will show you some methods to stablish the color of the path and the fill color of our shapes. For example with context.strokeStyle = "#ff0000"; we are setting red color for the line of our shapes. With context.fillStyle = "#0000ff"; we are setting the blue color to fill our shapes. Be carefull with these methods because maybe you fill with a color a region that you don't want to fill. To avoid this error, you can create a new draw with "context.beginPath()" and the select a color for the elements that you will draw from here.
All these processes look quite hard to draw something which looks fine, for that reason several companies are developing libraris to make our life easier and draw with canvas in an easier way. RGraph is a free library to create graphics and so in an easier way. I enclose here the code to create a columns graph that you can use in your websites. You will need to download the library from the RGraph website and copy the following code to your web:
- Use beginPath to start the draw.
- Use the method “lineTo” or “arc”.
- Optionally use closePath to finish our draw. This method joins the last point with the first one closing the draw.
- Finally, use stroke or fill to commit the drawing in the canvas. Stroke is used for the lines and fill is used when we want to fill the draw.
function draw() { var canvas = document.getElementById("board"); if (canvas.getContext) { var context = canvas.getContext("2d"); context.beginPath(); context.arc(75, 75, 50, 0, Math.PI * 2, true); //circle context.arc(75, 75, 35, 0, Math.PI, false); //mouth context.arc(60, 65, 5, 0, Math.PI * 2, true); //left eye context.arc(90, 65, 5, 0, Math.PI * 2, true); //right eye context.stroke(); } }
In the code we try to draw the classic smile by drawing each part of it. Unfortunately we are drawing over the canvas component like if we are not raising the pencil and it looks like this:
To avoid this effect we can use the method moveTo. With moveTo we raise the pencil over the canvas and we can get the effect of the following image.
function draw() { var canvas = document.getElementById("board"); if (canvas.getContext) { var context = canvas.getContext("2d"); context.beginPath(); context.arc(75, 75, 50, 0, Math.PI * 2, true); //circle context.moveTo(110, 75); context.arc(75, 75, 35, 0, Math.PI, false); //mouth context.moveTo(65, 65); context.arc(60, 65, 5, 0, Math.PI * 2, true); //left eye context.moveTo(95, 65); context.arc(90, 65, 5, 0, Math.PI * 2, true); //right eye context.stroke(); } }
Here, more methods to draw curves:
- quadraticCurveTo(cx1, cy1, x, y), used to draw Bézier curves with one control point,
- bezierCurveTo(cx1, cy1, cx2, cy2, x, y), used to draw Bézier curves with two control points,
- rect(x, y, width, height), to draw rectangles wich remains to a path.
Finally I will show you some methods to stablish the color of the path and the fill color of our shapes. For example with context.strokeStyle = "#ff0000"; we are setting red color for the line of our shapes. With context.fillStyle = "#0000ff"; we are setting the blue color to fill our shapes. Be carefull with these methods because maybe you fill with a color a region that you don't want to fill. To avoid this error, you can create a new draw with "context.beginPath()" and the select a color for the elements that you will draw from here.
All these processes look quite hard to draw something which looks fine, for that reason several companies are developing libraris to make our life easier and draw with canvas in an easier way. RGraph is a free library to create graphics and so in an easier way. I enclose here the code to create a columns graph that you can use in your websites. You will need to download the library from the RGraph website and copy the following code to your web:
window.onload = function () { var data = [280, 45, 133, 166, 84, 259, 266, 960, 219, 311, 67, 89]; var bar = new RGraph.Bar('myCanvas', data); bar.Set('chart.labels', ['Ene', 'Feb', 'Mar', 'Abr', 'May', 'Jun', 'Jul', 'Ago', 'Sep', 'Oct', 'Nov', 'Dic']); bar.Set('chart.gutter.left', 60); bar.Set('chart.gutter.bottom', 60); bar.Set('chart.title.yaxis', 'l/m2'); bar.Set('chart.title.xaxis', 'Months'); bar.Draw(); }
There are a lot of functions in canvas to add styles to our lines (lineWidht, lineCap, lineJoin), gradients (createLinearGradient, addColorStop, createRadialGradient), patterns (createPattern), draw images, draw text and so. Finally I want to show you what you can get with Canvas right now, these are a few of my favourites canvas projects:
- http://www.effectgames.com/demos/canvascycle/
- http://andrew-hoyer.com/experiments/cloth/
- http://29a.ch/sandbox/2010/box2d2/test.html
- https://developer.mozilla.org/en-US/demos/detail/ghostwriter-art-studio/launch
0 comments:
Post a Comment